layout: true class: animated fadeIn middle numbers .footnote[ `IPS-DEV` - N. Dubray - ENSIIE - 2024 - [:book:](../index.html) ] --- # Version control system: `git` .hcenter.w15[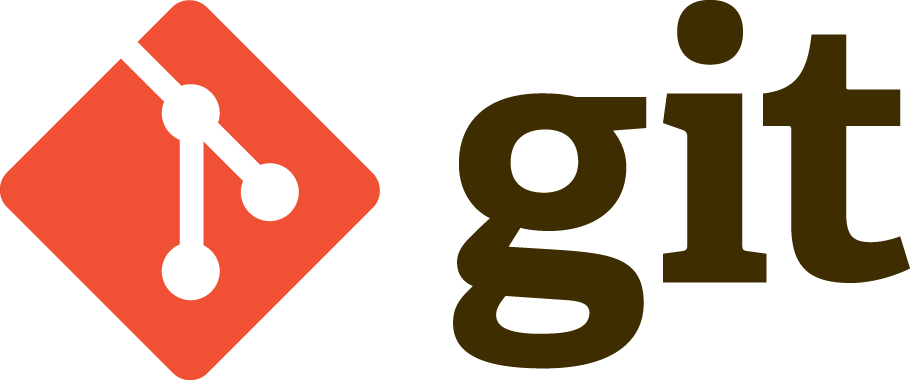] ## History * distributed version control system * created by **Linus Torvalds** in 2005 as free software (GPL v2) * initial goal: become the main VCS (Version Control System) of the **Linux kernel** * today: **#1 VCS** :v: ## Characteristics * strong support for non-linear development * distributed development * compatibility with existant systems and protocols * efficient handling of large projects * cryptographic authentication of history .block[ * Repository: [https://github.com/git/git](https://github.com/git/git) * Website: [https://git-scm.com](https://git-scm.com) * License: `LGPL-2.1` ] --- # `git` workflow .mermaid[ sequenceDiagram Remote-->>+Local repo: git clone/pull Note over Staged: Empty Local repo->>Files: git checkout Note over Files: Code... Files->>+Staged: git add/rm Staged->>-Local repo: git commit Note over Files: Code... Files->>+Staged: git add/rm Staged->>-Local repo: git commit Local repo-->>-Remote: git push ] --- # Creation of a `git` repo from scratch .hcenter[
] --- # Main `git` commands ## Configure `git` (should be done once) ```shell $ git config --global user.name "Your Name" $ git config --global user.email you@yourdomain.example.com ``` ## Create a local repo ```shell $ git init ``` ## Clone a remote repo (and create a local repo) ```shell $ git clone http://some.domain.com/path/to/repo.git ``` ## Get documentation ```shell $ # Example: documentation for command 'git clone' $ git help clone ``` --- class: top # Typical `git` workflow ## 1. clone or create repo ```shell $ git clone http://some.domain.com/path/to/repo.git ``` -- ## 2. make some changes ```shell $ vim README.md # create new file $ vim main.cpp # edit existing file $ rm toto.h # remove existing file ``` -- ## 3. **stage** changes ```shell $ git add main.cpp README.md $ git rm toto.h ``` -- ## 4. check staged changes ```shell $ git diff $ git status ``` -- ## 5. commit staged changes ```shell $ git commit # will open an editor to enter the commit message $ git commit -m "Commit message" ``` --- # `git` history ## View project history (CLI) ```shell $ git log $ git log --draw --oneline --color ```
--- # GUI tool `gitk` .hcenter.w90.shadow[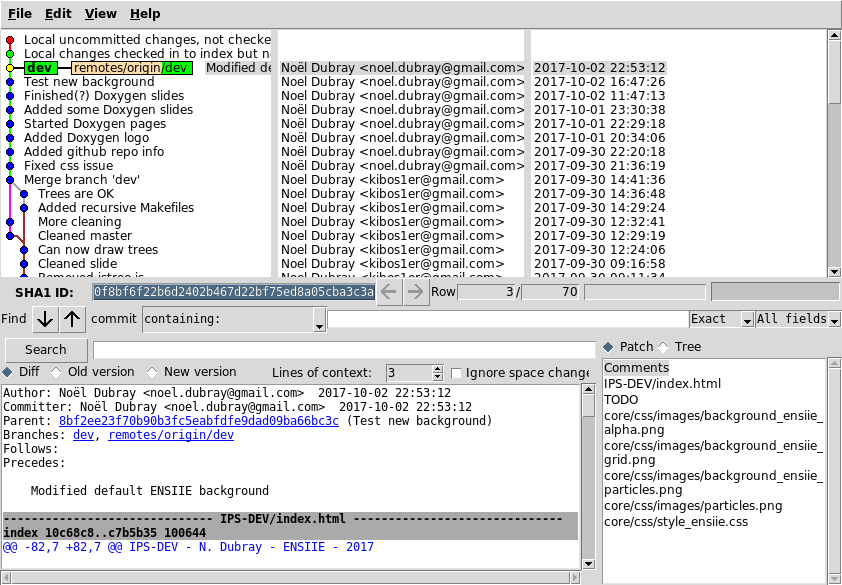] --- # GUI tool `gitg` .hcenter.w90.shadow[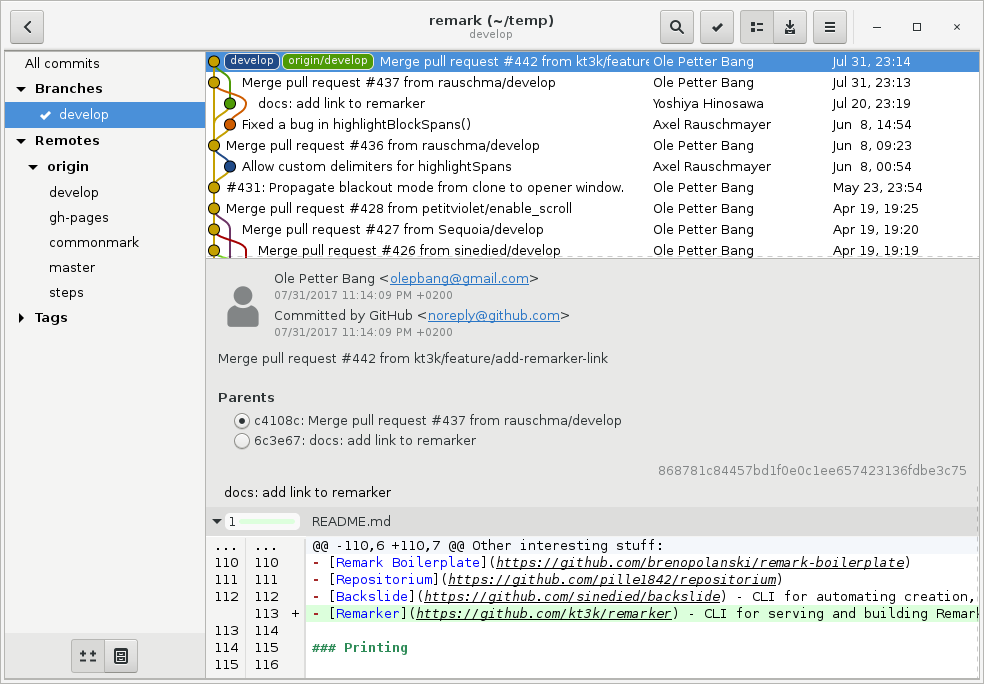] --- # `git` branches .row[ .column.middle.w45[ ## List branches ```shell $ git branch dev * main ``` ## Create a branch ```shell $ git branch dev ``` ## Switch to a branch ```shell $ git checkout dev ``` ## Create + switch to a branch ```shell $ git checkout -b dev ``` ## Merge a branch ```shell $ git merge dev ``` ## Delete a branch ```shell $ git branch -d dev ``` ] .column[
] ] --- class: top # How to use `git` branches .row[ .column.w45[ * SuperProg :copyright: is in state **v0.2.0** * you start working on a new functionnality by creating a branch and commiting on it ] .column[
] ] --- class: top # How to use `git` branches .row[ .column.w45[ * SuperProg :copyright: is in state **v0.2.0** * you start working on a new functionnality by creating a branch and commiting on it ```shell $ git checkout -b newfunc $ # some work $ git commit -m "Commit D" ... $ # some work $ git commit -m "Commit F" ``` ] .column[
] ] --- class: top # How to use `git` branches .row[ .column.w45[ * SuperProg :copyright: is in state **v0.2.0** * you start working on a new functionnality by creating a branch and commiting on it ```shell $ git checkout -b newfunc $ # some work $ git commit -m "Commit D" ... $ # some work $ git commit -m "Commit F" ``` * :warning: **issue #627 needs to be fixed** * you create a branch to fix the issue ] .column[
] ] --- class: top # How to use `git` branches .row[ .column.w45[ * SuperProg :copyright: is in state **v0.2.0** * you start working on a new functionnality by creating a branch and commiting on it ```shell $ git checkout -b newfunc $ # some work $ git commit -m "Commit D" ... $ # some work $ git commit -m "Commit F" ``` * :warning: **issue #627 needs to be fixed** * you create a branch to fix the issue ```shell $ git checkout master $ git checkout -b fixit $ # some work $ git commit -m "Commit G" ... $ # some work $ git commit -m "Fixed issue #627" ``` ] .column[
] ] --- class: top # How to use `git` branches .row[ .column.w45[ * SuperProg :copyright: is in state **v0.2.0** * you start working on a new functionnality by creating a branch and commiting on it ```shell $ git checkout -b newfunc $ # some work $ git commit -m "Commit D" ... $ # some work $ git commit -m "Commit F" ``` * :warning: **issue #627 needs to be fixed** * you create a branch to fix the issue ```shell $ git checkout master $ git checkout -b fixit $ # some work $ git commit -m "Commit G" ... $ # some work $ git commit -m "Fixed issue #627" ``` * you merge your fix in `master` ] .column[
] ] --- class: top # How to use `git` branches .row[ .column.w45[ * SuperProg :copyright: is in state **v0.2.0** * you start working on a new functionnality by creating a branch and commiting on it ```shell $ git checkout -b newfunc $ # some work $ git commit -m "Commit D" ... $ # some work $ git commit -m "Commit F" ``` * :warning: **issue #627 needs to be fixed** * you create a branch to fix the issue ```shell $ git checkout master $ git checkout -b fixit $ # some work $ git commit -m "Commit G" ... $ # some work $ git commit -m "Fixed issue #627" ``` * you merge your fix in `master` ```shell $ git checkout master $ git merge fixit $ git tag v0.2.1 # lightweight tag ``` ] .column[
] ] --- class: top # How to use `git` branches .row[ .column.w45[ * SuperProg :copyright: is in state **v0.2.1** * you can resume working on the new functionnality ] .column[
] ] --- class: top # How to use `git` branches .row[ .column.w45[ * SuperProg :copyright: is in state **v0.2.1** * you can resume working on the new functionnality ```shell $ git checkout newfunc $ # some work $ git commit -m "Commit J" ``` * when you are ready, merge the new functionnality in the `master` branch ] .column[
] ] --- class: top # How to use `git` branches .row[ .column.w45[ * SuperProg :copyright: is in state **v0.2.1** * you can resume working on the new functionnality ```shell $ git checkout newfunc $ # some work $ git commit -m "Commit J" ``` * when you are ready, merge the new functionnality in the `master` branch ```shell $ git checkout master $ git merge newfunc $ git tag v0.3.0 ``` ] .column[
] ] --- # Remote repositories .mermaid.w70.hcenter[ sequenceDiagram Remote-->>+Local repo: git clone/pull Local repo->>Files: git checkout Note over Files: Code... Files->>+Staged: git add/rm Staged->>-Local repo: git commit Local repo-->>-Remote: git push ] ## Create a bare repo ```shell $ git init --bare ``` ## Declare and sync from a remote repo ```shell $ git remote add farAway http://some.domain.com/path/to/repo.git $ git fetch farAway && git merge $ git pull farAway ``` --- # Conclusions on `git` .row[ .column.w40.middle[ ## To sum up... * very powerful tool, can be complex sometimes * **can work offline !** * rock-solid, very efficient * **decentralized !** * several source forges are using it (`github`, `bitbucket`, `gitlab`, etc...) * learn a few commands and start using it ! * several ways to use it, *follow the guidelines* * **the de-facto standard CVS** .hcenter.w50[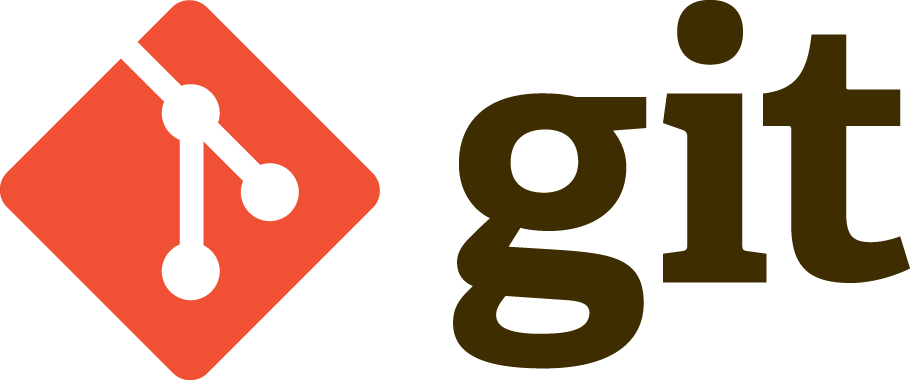] ] .column.w55[
] ]